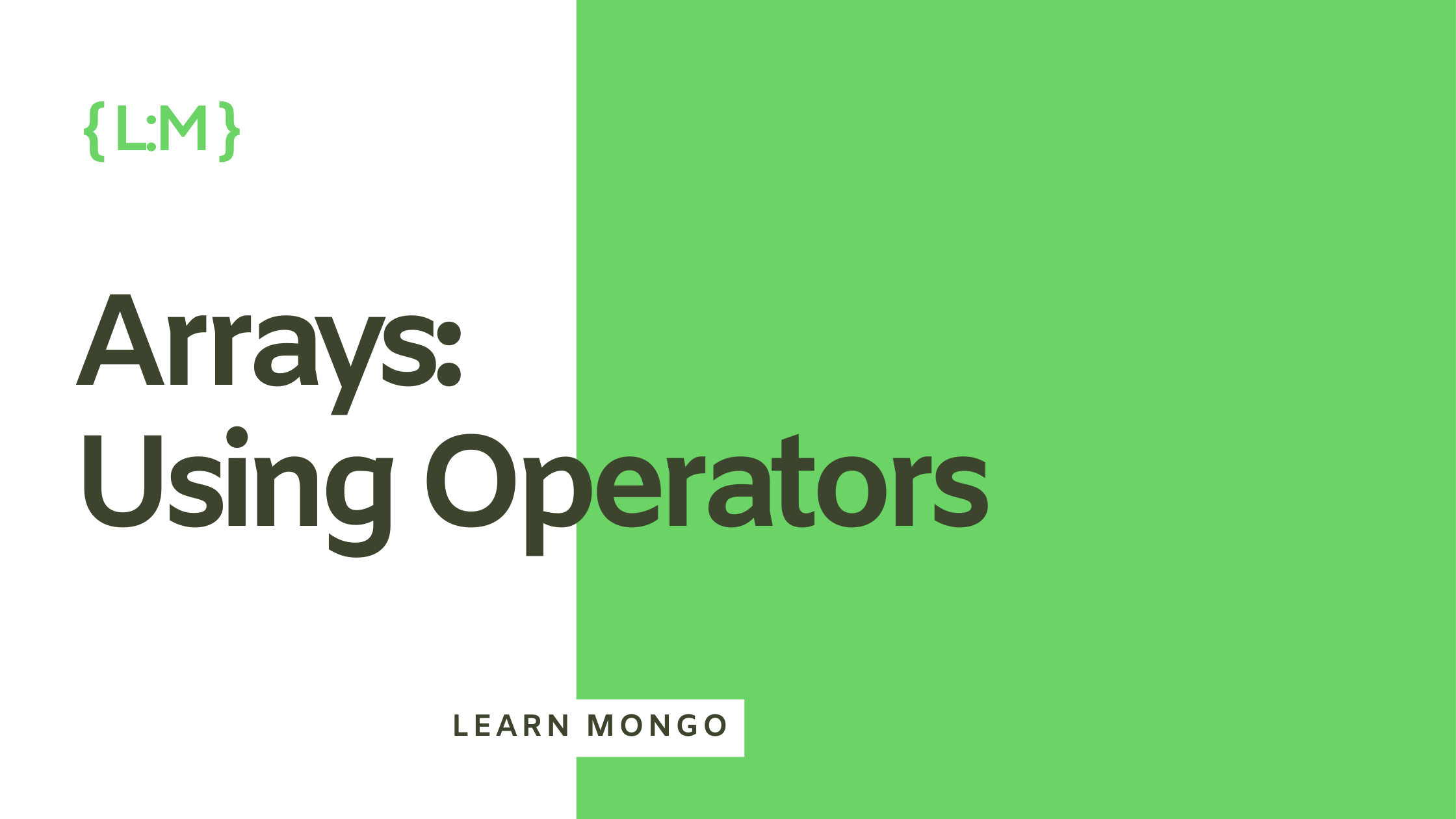
MongoDB offers a versatile and powerful way to work with array data using field query operators. Arrays are commonly used to store various types of data, and MongoDB’s array query operators allow you to manipulate and retrieve data from arrays efficiently. In this post, we’ll explore how to leverage some of these operators to make the most out of your array data.
Imagine a scenario where we have a cookbook collection containing recipes, each with a user rating
field representing an array of numbers ranging from 1
to 5
. The array might look something like this:
{
"rating": [3, 3, 4, 4, 5, 5, 3, 4, 4]
}
Let’s dive into some examples of how you can use MongoDB’s array query operators to extract meaningful insights from this data.
Finding Recipes with Specific Ratings
Suppose we’re interested in finding recipes that have any instance of a value lower than 4
in the rating
field array. In this case MongoDB’s $lt
(less than) operator comes in handy:
db.cookbook.find(
{ rating: { $lt: 4} }
).count();
This query will return the count of recipes that contain at least one rating less than 4
. Alternatively, we can achieve the same result using the $elemMatch
operator:
db.cookbook.find(
{ rating: { $elemMatch: { $lt: 4} } }
).count();
There are many more operators like this you can checkout in the docs.
Exploring Array Size
Another useful operator is $size
which allows us to determine if any of recipes have a specific number of items in the rating
field (and thus a certain number of ratings). For instance, to find recipes with only one or three ratings:
db.cookbook.find({ "rating": { $size: 1 } }).count();
db.cookbook.find({ "rating": { $size: 3 } }).count();
Retrieving Specific Elements in the Array
Awesome! Okay, so what if we want to retrieve recipes that have a rating of 5
as the first element in the rating
array? With MongoDB we can achieve this by specifying both the field and the array index using dot notation:
db.cookbook.find(
{ "rating.0": 5 },
{ "_id": 0, "title": 1, "rating": 1 }
);
This query returns recipes along with their titles and corresponding ratings, where the first element of the rating
array is 5
.
Additionally, let’s say we want to find recipes with a rating less than or equal to 3
:
db.cookbook.find(
{ "rating.0": { $lte: 3 } },
{ "_id": 0, "title": 1, "rating": 1 }
);
This query retrieves recipes with their titles and ratings, where the first element of the rating array is less than or equal to 3
.
Conclusion
MongoDB’s array field query operators provide a powerful toolkit for working with array data. Whether you’re searching for specific elements, checking array size, or querying based on certain conditions, these operators streamline your interactions with array fields. By mastering these techniques, you can unleash the full potential of MongoDB for handling complex data structures like arrays in your applications.
Remember to always refer to the MongoDB documentation for the latest information and best practices. Happy querying!