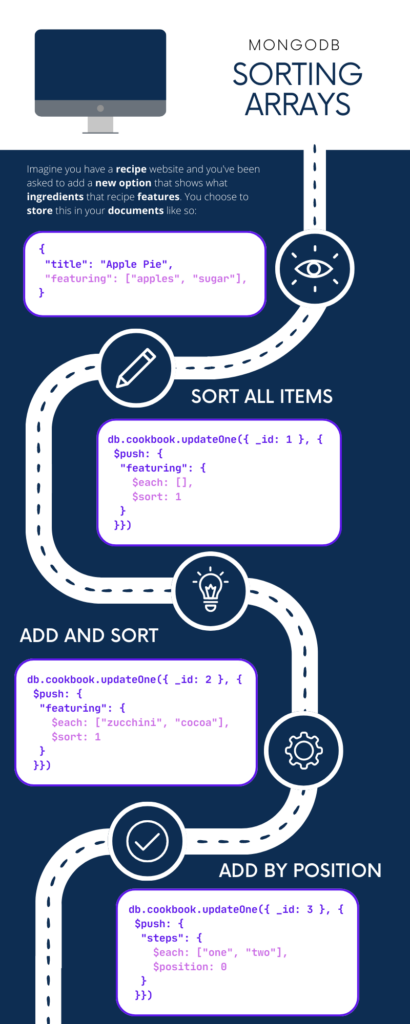
Arrays are a fundamental data structure in MongoDB, allowing you to store and manage collections of items. However, there are times when the specific order of items within an array is very import. MongoDB provides a powerful tool for achieving this precision: the $sort
operator.
In this post, we’ll delve into how to leverage the $sort
operator to maintain and manipulate the order of array elements during inserts and updates.
The Importance of Array Order
Consider scenarios where the sequence of items within an array plays a crucial role. Let’s explore how the $sort
operator can help us maintain this order, using an illustrative example involving a field named featuring
.
Imagine having an array of items like this:
{
"featuring": ["apples", "cinnamon", "sugar"]
}
Now, let’s say we want to add two new items, “zucchini” and “blueberries,” while ensuring that the original order is preserved (in this case, alphabetical). This is where the $sort
operator comes into play.
db.cookbook.updateOne(
{ _id: 1 },
{
$push: {
"featuring": {
$each: ["zucchini", "blueberries"],
$sort: 1
}
}
}
)
After executing this operation, our array would be transformed as follows:
{
"featuring": ["apples", "blueberries", "cinnamon", "sugar", "zucchini"]
}
In MongoDB, using $sort
with a value of 1
indicates ascending order, and -1
signifies descending order. It’s also worth noting that an empty array []
can be employed with $sort
when the sole intention is to rearrange items without modifying them.
db.cookbook.updateOne(
{ _id: 1 },
{
$push: {
"featuring": {
$each: [],
$sort: 1
}
}
}
)
Maintaining Specific Order
In some cases, you may desire to ensure that the most recently added item always occupies a specific position within an array. This can be achieved using the $position
operator in conjunction with the $each
operator.
For instance, let’s assume we want to add “one” and “two” to a steps
array, always positioning them at the beginning:
db.cookbook.updateOne(
{ _id: 1 },
{
$push: {
"steps": {
$each: ["one", "two"],
$position: 0
}
}
}
)
With $position
set to 0
, these new items will be added to the start of the array. Conversely, a value of -1
would insert the items just before the last element in the array. By default, the $push
operation appends items to the end of the array.
In conclusion, mastering the $sort
and $position
operators in MongoDB provides you with powerful tools to meticulously manage the order of array elements. Whether it’s preserving a specific sequence or controlling where new items are placed, these operators empower you to manipulate complex data with elegance and precision.
By effectively utilizing these features, you can ensure that your data structures align with your application’s requirements, enhancing performance and overall functionality.