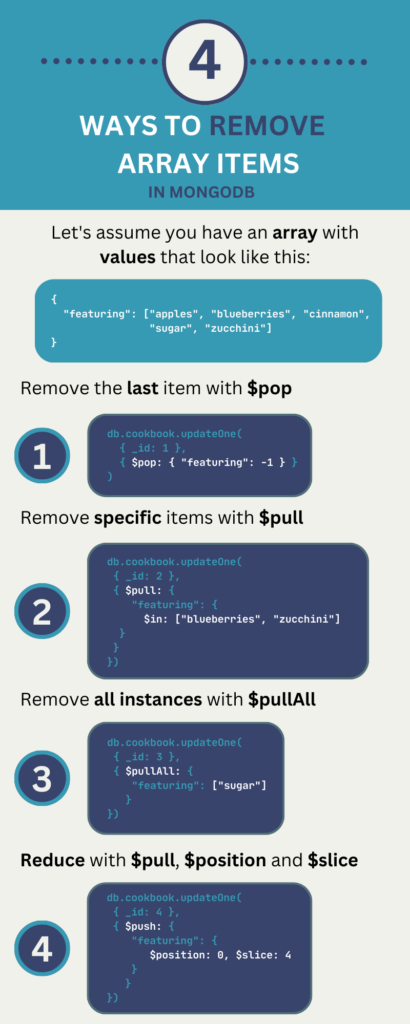
In the world of MongoDB, arrays bring order to chaos and enable the efficient organization of data. Yet, there come times when the need arises to prune or refine the contents of an array.
In this exploration, we’ll delve into the mechanisms behind removing elements from arrays, making your data management journey smoother than ever.
Trimming the Edges of Data Arrays
Picture this: You’re managing a culinary website, and you want to showcase a preview of the most recent user ratings. To facilitate this, you create a field called last_four_ratings
to house the last four user ratings for each recipe. Maybe your array field looks like this:
{
"last_four_ratings": [2, 3, 3, 4]
}
So what do you do when a recipe gets more than four ratings? How do you make sure you only have the last four in your array?
Enter the $slice
operator, a formidable tool for precisely controlling the size of an array during updates. To seamlessly add a new (hopefully) 5-star ratings, you can use the $push
operation along with $slice
:
db.cookbook.updateOne(
{ _id: 1 },
{
$push: {
"last_four_ratings": {
$each: [5, 5],
$position: 0,
$slice: 4
}
}
}
)
By employing $each
with two values of 5
and setting $slice
to 4
, you ensure the array retains only four elements after the update. The $position
of 0
adds the new values to the front of the array. The final result:
{
"last_four_ratings": [5, 5, 2, 3]
}
Beyond $slice
, MongoDB offers two other commonly used methods for removing elements: $pop
for removing the first or last element, and $pull
for removing items based on specified criteria.
Orchestrating Precise Removals
Suppose you want to refine your recipe documents by focusing on a subset of featured ingredients. If your featuring
array looks like this:
{
"featuring": ["apples", "blueberries", "cinnamon", "sugar", "zucchini"]
}
To remove the last item, you can utilize $pop
:
db.cookbook.updateOne(
{ _id: 1 },
{ $pop: { "featuring": -1 } }
)
The outcome:
{
"featuring": ["apples", "blueberries", "cinnamon", "sugar"]
}
For more targeted removals, $pull
comes into play. To eliminate specific ingredients, construct a query using $pull
:
db.cookbook.updateOne(
{ _id: 1 },
{ $pull: { "featuring": { $in: ["blueberries", "zucchini"] } } }
)
This query removes “blueberries” and “zucchini” from the array, leaving:
{
"featuring": ["apples", "cinnamon", "sugar"]
}
And don’t forget the power of $pullAll
– it nimbly erases all instances of a value from the array without requiring a query:
db.cookbook.updateOne(
{ _id: 1 },
{ $pullAll: { "featuring": ["sugar"] } }
)
The aftermath:
{
"featuring": ["apples", "cinnamon"]
}
Beyond the Basics
These array operators barely scratch the surface of MongoDB’s capabilities. As your data management journey unfolds, consider exploring more advanced methods and array operations. For an in-depth understanding of array manipulation in MongoDB, consult the official documentation to discover a world of possibilities.